- Home
- Programming
- OCA Java SE8
41.
Of the types double, int, and short, how many could fill in the blank to have this code
output 0?
public static void main(String[] args) {
_______defaultValue;
System.out.println(defaultValue);
}
public static void main(String[] args) {
_______defaultValue;
System.out.println(defaultValue);
}
- A.None
- B.One
- C.Two
- D.Three
- Answer & Explanation
- Report
Answer : [A]
Explanation :
Explanation :
Since defaultValue is a local variable, it is not automatically initialized. That means the code will not compile with any type. Therefore, Option A is correct. If this was an instance variable, Option C would be correct as int and short would be initialized to 0 while double would be initialized to 0.0. |
42.
What is true of the finalize() method?
- A.It may be called zero or one times.
- B.It may be called zero or more times.
- C.It will be called exactly once.
- D.It may be called one or more times.
- Answer & Explanation
- Report
Answer : [A]
Explanation :
Explanation :
The finalize() method may not be called, such as if your program crashes. However, it is guaranteed to be called no more than once. |
43.
Which of the following is not a wrapper class?
- A.Double
- B.Integer
- C.Long
- D.String
- Answer & Explanation
- Report
Answer : [D]
Explanation :
Explanation :
String is a class, but it is not a wrapper class. In order to be a wrapper class, the class must have a one-to-one mapping with a primitive. |
44.
Suppose you have the following code. Which of the images best represents the state of
the references right before the end of the main method, assuming garbage collection
hasn’t run?
1: public class Link {
2: private String name;
3: private Link next;
4: public Link(String name, Link next) {
5: this.name = name;
6: this.next = next;
7: }
8: public void setNext(Link next) {
9: this.next = next;
10: }
11: public Link getNext() {
12: return next;
13: }
14: public static void main(String... args) {
15: Link link1 = new Link("x", null);
16: Link link2 = new Link("y", link1);
17: Link link3 = new Link("z", link2);
18: link2.setNext(link3);
19: link3.setNext(link2);
20: link1 = null;
21: link3 = null;
22: }
23: }
1: public class Link {
2: private String name;
3: private Link next;
4: public Link(String name, Link next) {
5: this.name = name;
6: this.next = next;
7: }
8: public void setNext(Link next) {
9: this.next = next;
10: }
11: public Link getNext() {
12: return next;
13: }
14: public static void main(String... args) {
15: Link link1 = new Link("x", null);
16: Link link2 = new Link("y", link1);
17: Link link3 = new Link("z", link2);
18: link2.setNext(link3);
19: link3.setNext(link2);
20: link1 = null;
21: link3 = null;
22: }
23: }
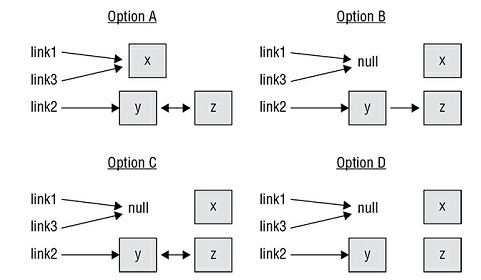
- A.Option A
- B.Option B
- C.Option C
- D.Option D
- Answer & Explanation
- Report
Answer : [C]
Explanation :
Explanation :
Lines 15–17 create the three objects. Lines 18–19 change the references so link2 and link3 point to each other. The lines 20–21 wipe out two of the original references. This means the object with name as x is inaccessible. |
45.
Which type can fill in the blank?
_______ pi = 3.14;
_______ pi = 3.14;
- A.byte
- B.float
- C.double
- D.short
- Answer & Explanation
- Report
Answer : [C]
Explanation :
Explanation :
Options A and D are incorrect because byte and short do not store values with decimal points. Option B is tempting. However, 3.14 is automatically a double. It requires casting to float or writing 3.14f in order to be assigned to a float. Therefore, Option C is correct. |